Useful things of modern C++
Modern C++ is (in my understanding) everything that starts with the C++11 standard. Which is already 9 years old. And just recently I decided to brush up on my knowledge of this wonderful PL, for which I found and collected all the features of C++11, C++14 and C++17 that are interesting for me personally. The result is this memo, which is needed primarily for me to systematize knowledge and as a small reference book.
Let's start with what's new in C++11.
1. https://en.cppreference.com/w/cpp/language/static_assert
Since C++11 it is possible to use static_assert (and in C++17 this mechanism is simplified) for compile-time checks. And rather “smart” development environments (for example, Visual Studio 2019) do such checks at the stage of editing the source code.

2. https://en.cppreference.com/w/cpp/language/auto
Automatic type deduction greatly simplifying, for example, looping through STL containers:

3. https://en.cppreference.com/w/cpp/language/function#Deleted_functions
You can “disable” certain functions of a class, for example, the default constructor.

4. https://en.cppreference.com/w/cpp/language/final
You can “finalize” a class or function, making overriding or inheritance impossible:

5. https://en.cppreference.com/w/cpp/language/override
The override keyword can be used to explicitly indicate the intent to override a function. Tighter control over the inheritance mechanism is of course useful.

6. https://en.cppreference.com/w/cpp/language/move_assignment
https://en.cppreference.com/w/cpp/language/move_constructor
https://en.cppreference.com/w/cpp/utility/move
No more temporary objects!
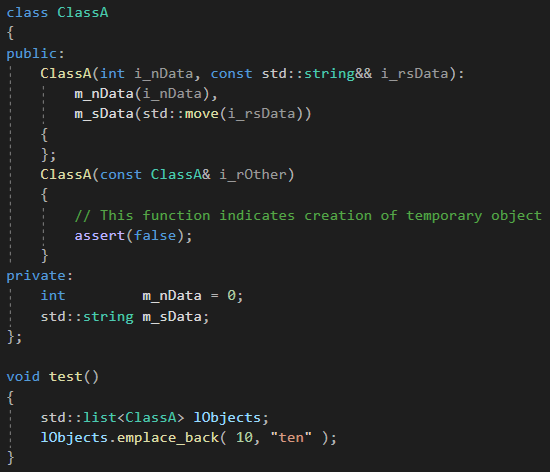
7. https://en.cppreference.com/w/cpp/language/enum
The enumeration type has become more useful due to the ability to explicitly set the type of a value and restrict access to its elements by the requirement of explicitly specify enumeration name.

8. https://en.cppreference.com/w/cpp/language/user_literal
User-defined literals allow you to make your code more readable by hiding the function call behind a nice-looking declaration.
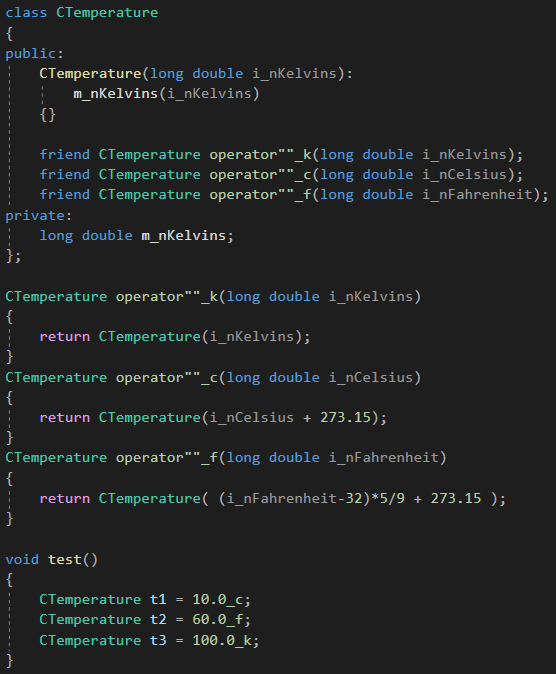
9. https://en.cppreference.com/w/cpp/language/list_initialization
Initialization list simplifies initialization of STL containers (and may be used for user class).

10. https://en.cppreference.com/w/cpp/language/initializer_list#Delegating_constructor
Now one constructor can call another.
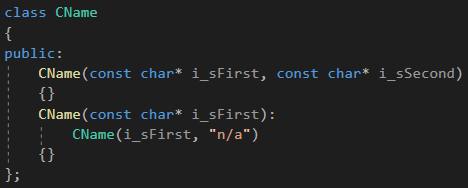
11. https://en.cppreference.com/w/cpp/language/nullptr
Finally, no more NULL macros and stuff like that. There is now a special data type for this.
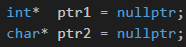
12. https://en.cppreference.com/w/cpp/language/types#Character_types
https://en.cppreference.com/w/cpp/language/string_literal
Enhanced Unicode support at the level of language types and literals:

13. https://en.cppreference.com/w/cpp/language/lambda
One of the most useful innovations. Allows you to declare functions exactly where they are needed. Although I would not abuse this possibility.

14. https://en.cppreference.com/w/cpp/language/range-for
Now, instead of using indices and iterators, you can get by with this simple and neat construction:

15. https://en.cppreference.com/w/cpp/header/array
More safe and convenient replacement for C-style arrays.

16. https://en.cppreference.com/w/cpp/header/random
Much more advanced random number generation possibilities.

17. https://en.cppreference.com/w/cpp/header/regex
Finally, regular expressions are part of the standard library!

18. https://en.cppreference.com/w/cpp/thread
https://en.cppreference.com/w/cpp/header/thread
https://en.cppreference.com/w/cpp/header/mutex
https://en.cppreference.com/w/cpp/header/condition_variable
https://en.cppreference.com/w/cpp/header/future
https://en.cppreference.com/w/cpp/atomic
Multithreading is now supported by the standard library. A very useful innovation.

I found two interesting innovations in C++14:
19. https://en.cppreference.com/w/cpp/language/integer_literal
Binary literals allow you to write numbers in binary form:

20. Ability to use a separator in numeric literals:

More cool stuff in C++17 (plus the previously mentioned simplification for static_assert).
21. https://en.cppreference.com/w/cpp/language/attributes
An attribute indicating that the presence of an unused entity is not an error. No more UNUSED macros.

22. https://en.cppreference.com/w/cpp/language/namespace
Declaration of nested namespaces is simplified:

23. https://en.cppreference.com/w/cpp/utility/any
A special data type can store the value of any type:

24. https://en.cppreference.com/w/cpp/header/variant
You no longer need to reinvent your variant type as it appeared in the standard library.
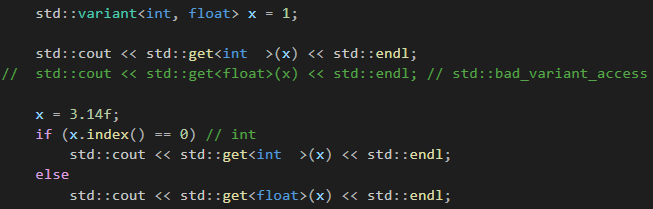
25. https://en.cppreference.com/w/cpp/header/optional
Optional function arguments and return values.

26. https://en.cppreference.com/w/cpp/header/string_view
Better, safer alternative to C-style strings.

27. https://en.cppreference.com/w/cpp/header/charconv
Another (seemingly faster) way to convert text to numbers and vice versa.
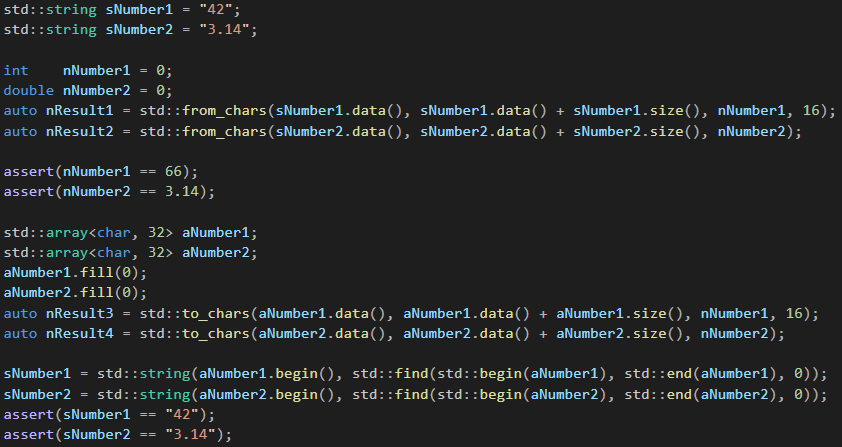
28. https://en.cppreference.com/w/cpp/algorithm/clamp
A simple function to limit a number on the lower and upper bounds.

29. https://en.cppreference.com/w/cpp/header/filesystem
Full-blown support of the file system. A lot of useful things, for example - getting a list of files in a directory.

Let's start with what's new in C++11.
1. https://en.cppreference.com/w/cpp/language/static_assert
Since C++11 it is possible to use static_assert (and in C++17 this mechanism is simplified) for compile-time checks. And rather “smart” development environments (for example, Visual Studio 2019) do such checks at the stage of editing the source code.
2. https://en.cppreference.com/w/cpp/language/auto
Automatic type deduction greatly simplifying, for example, looping through STL containers:
3. https://en.cppreference.com/w/cpp/language/function#Deleted_functions
You can “disable” certain functions of a class, for example, the default constructor.
4. https://en.cppreference.com/w/cpp/language/final
You can “finalize” a class or function, making overriding or inheritance impossible:
5. https://en.cppreference.com/w/cpp/language/override
The override keyword can be used to explicitly indicate the intent to override a function. Tighter control over the inheritance mechanism is of course useful.
6. https://en.cppreference.com/w/cpp/language/move_assignment
https://en.cppreference.com/w/cpp/language/move_constructor
https://en.cppreference.com/w/cpp/utility/move
No more temporary objects!
7. https://en.cppreference.com/w/cpp/language/enum
The enumeration type has become more useful due to the ability to explicitly set the type of a value and restrict access to its elements by the requirement of explicitly specify enumeration name.
8. https://en.cppreference.com/w/cpp/language/user_literal
User-defined literals allow you to make your code more readable by hiding the function call behind a nice-looking declaration.
9. https://en.cppreference.com/w/cpp/language/list_initialization
Initialization list simplifies initialization of STL containers (and may be used for user class).
10. https://en.cppreference.com/w/cpp/language/initializer_list#Delegating_constructor
Now one constructor can call another.
11. https://en.cppreference.com/w/cpp/language/nullptr
Finally, no more NULL macros and stuff like that. There is now a special data type for this.
12. https://en.cppreference.com/w/cpp/language/types#Character_types
https://en.cppreference.com/w/cpp/language/string_literal
Enhanced Unicode support at the level of language types and literals:
13. https://en.cppreference.com/w/cpp/language/lambda
One of the most useful innovations. Allows you to declare functions exactly where they are needed. Although I would not abuse this possibility.
14. https://en.cppreference.com/w/cpp/language/range-for
Now, instead of using indices and iterators, you can get by with this simple and neat construction:
15. https://en.cppreference.com/w/cpp/header/array
More safe and convenient replacement for C-style arrays.
16. https://en.cppreference.com/w/cpp/header/random
Much more advanced random number generation possibilities.
17. https://en.cppreference.com/w/cpp/header/regex
Finally, regular expressions are part of the standard library!
18. https://en.cppreference.com/w/cpp/thread
https://en.cppreference.com/w/cpp/header/thread
https://en.cppreference.com/w/cpp/header/mutex
https://en.cppreference.com/w/cpp/header/condition_variable
https://en.cppreference.com/w/cpp/header/future
https://en.cppreference.com/w/cpp/atomic
Multithreading is now supported by the standard library. A very useful innovation.
I found two interesting innovations in C++14:
19. https://en.cppreference.com/w/cpp/language/integer_literal
Binary literals allow you to write numbers in binary form:
20. Ability to use a separator in numeric literals:
More cool stuff in C++17 (plus the previously mentioned simplification for static_assert).
21. https://en.cppreference.com/w/cpp/language/attributes
An attribute indicating that the presence of an unused entity is not an error. No more UNUSED macros.
22. https://en.cppreference.com/w/cpp/language/namespace
Declaration of nested namespaces is simplified:
23. https://en.cppreference.com/w/cpp/utility/any
A special data type can store the value of any type:
24. https://en.cppreference.com/w/cpp/header/variant
You no longer need to reinvent your variant type as it appeared in the standard library.
25. https://en.cppreference.com/w/cpp/header/optional
Optional function arguments and return values.
26. https://en.cppreference.com/w/cpp/header/string_view
Better, safer alternative to C-style strings.
27. https://en.cppreference.com/w/cpp/header/charconv
Another (seemingly faster) way to convert text to numbers and vice versa.
28. https://en.cppreference.com/w/cpp/algorithm/clamp
A simple function to limit a number on the lower and upper bounds.
29. https://en.cppreference.com/w/cpp/header/filesystem
Full-blown support of the file system. A lot of useful things, for example - getting a list of files in a directory.
Comments
Post a Comment